You may work with one partner on this lab. Only one member of the group needs to submit the lab, but include both of your names in a comment in the
test_rgb.cpp
In this lab you will practice working with an RGB image using the RGBImage class.
working with RGB components
Write code to split a PNG image into four sub-images showing the red, green, blue, and grey components separately. The grey component is computed by taking the average of the r,g,b components for a given pixel. An example is shown below
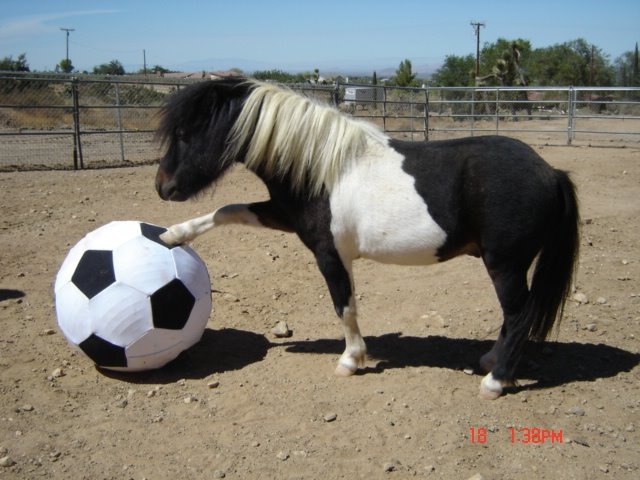
img found here.
Nothing too tricky here, but note that each sub-image is 1/4 the size of the original. You can decide how to sample pixels in the original image to create the sub-image. One possibility is to take the upper left pixel of each 2x2 block of four pixels in the original image.
Bresenham's line algorithm
Add a method
line(int x1, int y1, int x2, int y, const RGBColor& clr) to the RGBImage class that draws a line between two endpoints using Bresenham's line algorithm as discussed in class. Note we only handled the case where the slope of the line was between 0 and 1 and when
x1 < x2. You should handle other cases appropriately and test that your code works for these cases.
Do not make heavy use of copy paste to handle special cases. Use symmetry.
Create images
Create a least one image that displays the correct handling of special cases of Bresenham's algorithm, an image that displays the separation into four sub images and an image of your own design.
If all is working well, you should be able to test your code using
cumin[labs]$ ls
01/ CMakeLists.txt
cumin[labs]$ mkdir build
cumin[labs]$ cd build/
cumin[build]$ cmake ..
cumin[build]$ make
cumin[build]$ cd 01
cumin[01]$ ./test_rgb
Optional Extensions
These extensions are entirely optional and should not be attempted until the required components are complete.
- Try Wu's line algorithm for anti-aliased lines. You may use floating point math here.
- Allow the line drawing algorithm to draw lines of various (integer) widths.
- Add some image effects for manipulating RGBImages. Examples might include blur or posterize.
Submit
Once you are satisfied with your programs, hand them in by typing
handin40 at the unix prompt.
You may run
handin40 as many times as you like, and only the
most recent submission will be recorded. This is useful if you realize
after handing in some programs that you'd like to make a few more
changes to them.