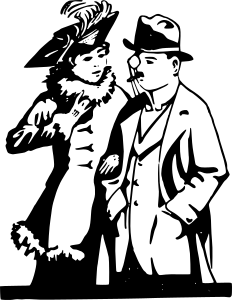
When writing programs, it is in your best interest to keep everything tidy. By following consistent style guidelines, you will find it easier to read and edit your code (and, for pair labs, your partner will as well). Here are some examples of good style when writing C++. Please remember to refer to the lab write-ups to know what is required for each assignment.
You should pick meaningful variable names.
// Good int* pixels = new int[size]; // Bad int* p = new int[size];
You should use correct and consistent indentation. Lines of code
within a block (that is, surrounded by {
and }
) should be
indented two spaces further than the lines surrounding them.
// Good if (condition) { cout << "Test" << endl; } // Bad if (condition) { cout << "Test" << endl; }
You should use a block whenever possible, even if it’s not necessary. This helps you avoid subtle or messy bugs in the future.
// Good if (condition) { cout << "Something" << endl; } // Bad if (condition) cout << "Something" << endl;
Any new methods or fields in your header files should have comments
explaining their purpose and behavior. You are permitted to omit
documentation for methods that are inherited from other classes;
that is, if your class has a foo
method because its superclass has
a foo
method, you don’t need to document that method.
// Good public: /** * Saves the image represented by this object to a file. * @param filename The name of the file to save. * @return The number of pixels in the saved image. * @throws runtime_error If the image could not be saved due to an I/O error. */ int save(std::string filename);
// Bad public: int save(std::string filename);
Your method/field documentation does not have to be in the format above, but you must describe the method’s behavior, its parameters, its return value, and any exceptions that it may throw. (If you’re indifferent, the above syntax is a good one to know; it’s a de facto standard used by Javadoc, Doxygen, and other tools that automatically process source code comments into other formats like searchable webpages.)