1. Goals for this week:
-
Learn more about how C program can map to assembly program and an executable binary program.
-
Practice writing and compiling Assembly (ASM) code.
-
Use the ASM visualizer to trace through Assembly code.
-
Introduction to Lab 5.
2. Starting Point Code
Start by creating a week06
in your cs31/WeeklyLabs
subdirectory
and copying over some files:
$ cd ~/cs31/WeeklyLabs
$ mkdir week06
$ cd week06
$ pwd
/home/you/cs31/WeeklyLabs/week06
$ cp ~sukrit/public/cs31/week06/* ./
$ ls
Makefile dosomething.s dosomething.c prog.c simpleops.c
3. Using gcc to generate assembly
Let’s try out gcc
to build assembly files and .o
files and look at the results. Open up simpleops.c
.
We are going to look at how to use gcc
to create an assembly version of
this file, how to create an object .o
file, and how to examine its
contents. We are also going to look at how to used gdb
to see the assembly
code and to step through the execution of individual instructions.
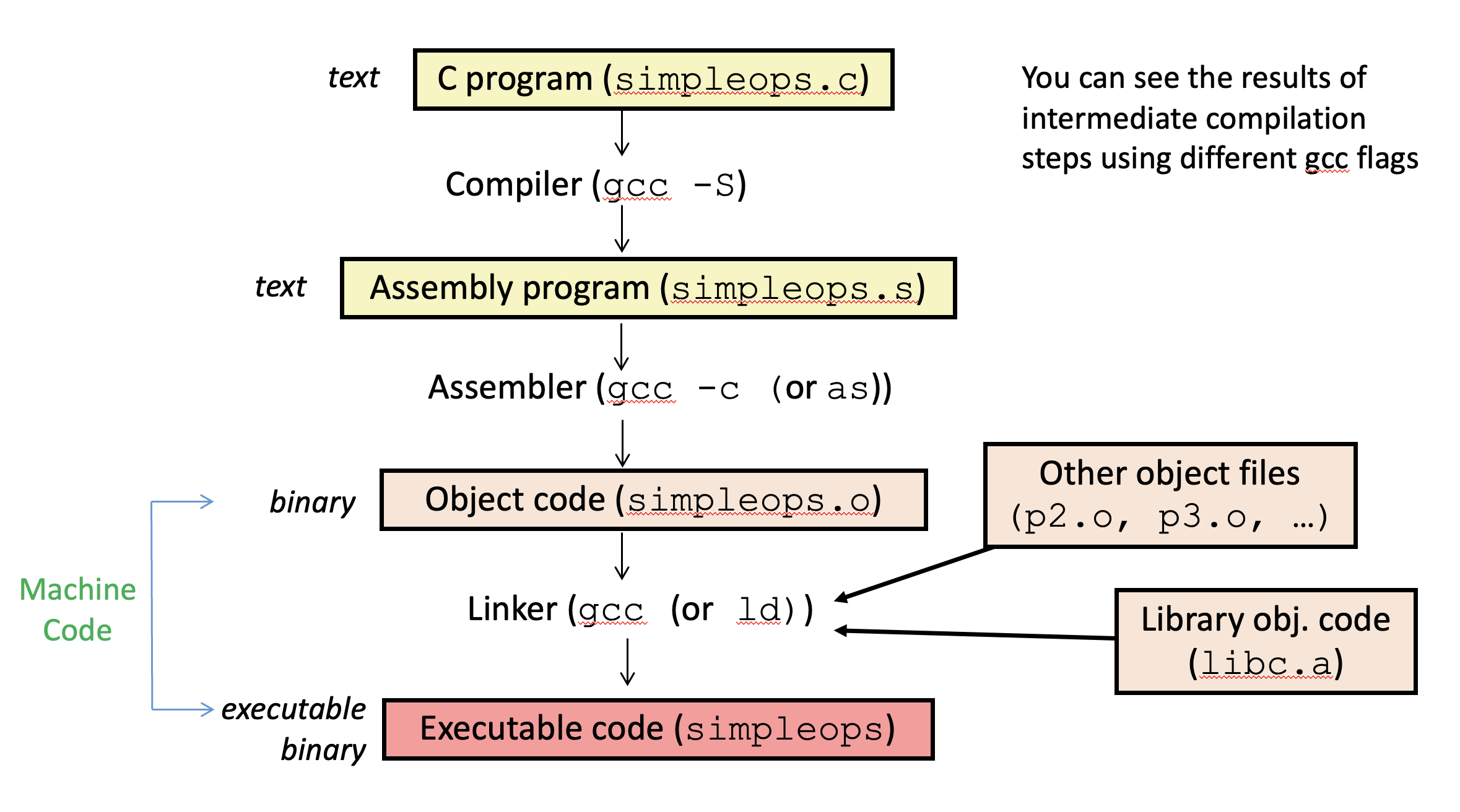
If you open the Makefile
, you can see the rules for building .s
, .o
and executable files from simpleops.c
.
$ gcc -S simpleops.c # just runs the assembler to create a .s text file
$ gcc -c simpleops.s # compiles to a relocatable object binary file (.o)
$ gcc -o simpleops simpleops.o # creates an executable file
To see the machine code and assembly code mappings in the .o
file:
$ objdump -d simpleops.o
You can compare this to the assembly file:
$ cat simpleops.s
4. Compiling and Running Assembly Code on Lab Machines
As part of Lab 5, you’ll be writing two small assembly
programs. You may (hopefully) find it helpful to use the
{visualizerurl}[assembly visualization tool] when working on it (discussed next),
but ultimately you’ll need to submit a file for grading.
You can compile and run assembly programs (whose file names end in .s
) like this:
$ gcc -o prog prog.c dosomething.s $ make # or just type make to compile $ ./prog
The dosomething.s
file in today’s starter code demonstrates an example of
compiling an assembly program like. For the lab, we will provide a
Make
file that will compile your assembly and C code for you.
5. Writing x86_64 Assembly Code
This semester, we’re going to be learning a assembly visualization tool that was developed here at Swarthmore. You can use this tool whenever you would like to trace small snippets of x86_64 assembly language. For this in-lab exercise, we’d like you to test out two examples in the visualizer.
As you go, you may find it helpful to refer to the x86_64 instruction reference sheet.
5.1. Task 1: Code Tracing
For the first task, trace through the following assembly code and figure out what it is doing. Work with a neighbor or lab partner to step through the code and describe to each other what’s happening. Roughly speaking, what would equivalent C code look like?
Next, paste this code into an Assembly Visualizer window (check that you have the "Arithmetic" view and not the "Function" view window to copy code into), then press the submit button:
subq $16, %rsp movq $10, -8(%rbp) movq -8(%rbp), %rax movq $5, %rdx addq %rax, %rdx cmp %rax, %rdx jg .L1 subq $3, %rax movq %rax, -16(%rbp) jmp .L2 .L1: movq $0xff, -16(%rbp) .L2: movq -16(%rbp), %rax addq $16, %rsp
5.2. Task 2: Writing Assembly
For the second task, you will write assembly code to compute the sum
of the values 1 to 5 using a loop. For example, you could try converting the
following while loop to x86_64 (you can use stack locations
%rbp
-8 and %rbp
-16 or any of the general purpose registers for the
variables i and sum):
i = 1;
sum = 0;
while (i <= 5) {
sum = sum + i;
i++;
}
Work with a neighbor or lab partner to write the C goto equivalent
of this code in a text editor, fllowed by the x86_64 assembly equivalent of
this while
loop in the "Arithmetic" view.
For reasons we haven’t explained in class yet, you should include the following two lines as the first and last lines of your solution:
subq $16, %rsp
... # your solution goes here
addq $16, %rsp
We strongly suggest that you write your code in your favorite text editor and then copy it into the visualization tool when you’re ready to run it in case you accidentally refresh your browser window. |
6. Lab 5
Finally, let’s take a look at Lab 5.
7. Handy References
-
gdb for IA32 assembly debugging IA32 gdb debugging guide
-
GDB for Assembly (from the gdb Guide). (assembly debugging and
x
command) -
Sections 3.2 and 3.5 of textbook (assembly debugging, print, display, info and
x
commands) -
Tools for examining phases of compiling and running C programs